Using Go Modules Tutorial
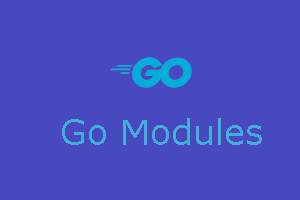
What is Go Module?
A module is a collection of Go packages stored in a file tree with a go.mod file at its root. The go.mod
file defines the module’s module path, which is also the import path used for the root directory, and its dependency requirements, which are the other modules needed for a successful build. Each dependency requirement is written as a module path and a specific semantic version.
This post is part 1 in a series.
- Part 1 — Using Go Modules (this post)
- Part 2 — Migrating To Go Modules
- Part 3 — Publishing Go Modules
Creating a new module in GO
Create a new, empty directory somewhere outside $GOPATH/src
, cd into that directory, and then create a new source file, hello.go:
1 2 3 4 5 | package hello func Hello() string { return "Hello, world." } |
Adding a dependency in Go module
The primary motivation for Go modules was to improve the experience of using (that is, adding a dependency on) code written by other developers.
Let’s update our hello.go to import rsc.io/quote
and use it to implement Hello:
1 2 3 4 5 6 7 | package hello import "rsc.io/quote" func Hello() string { return quote.Hello() } |
Conclusion
Go modules are the future of dependency management in Go. Module functionality is now available in all supported Go versions (that is, in Go 1.11 and Go 1.12).
This post introduced these workflows using Go modules:
go mod init
creates a new module, initializing the go.mod file that describes it.go build
,go test
, and other package-building commands add new dependencies to go.mod as needed.go list -m all
prints the current module’s dependencies.- go get changes the required version of a dependency (or adds a new dependency).
- go mod tidy removes unused dependencies.
Usefull Link
- Part 2 — Migrating To Go Modules
- Part 3 — Publishing Go Modules